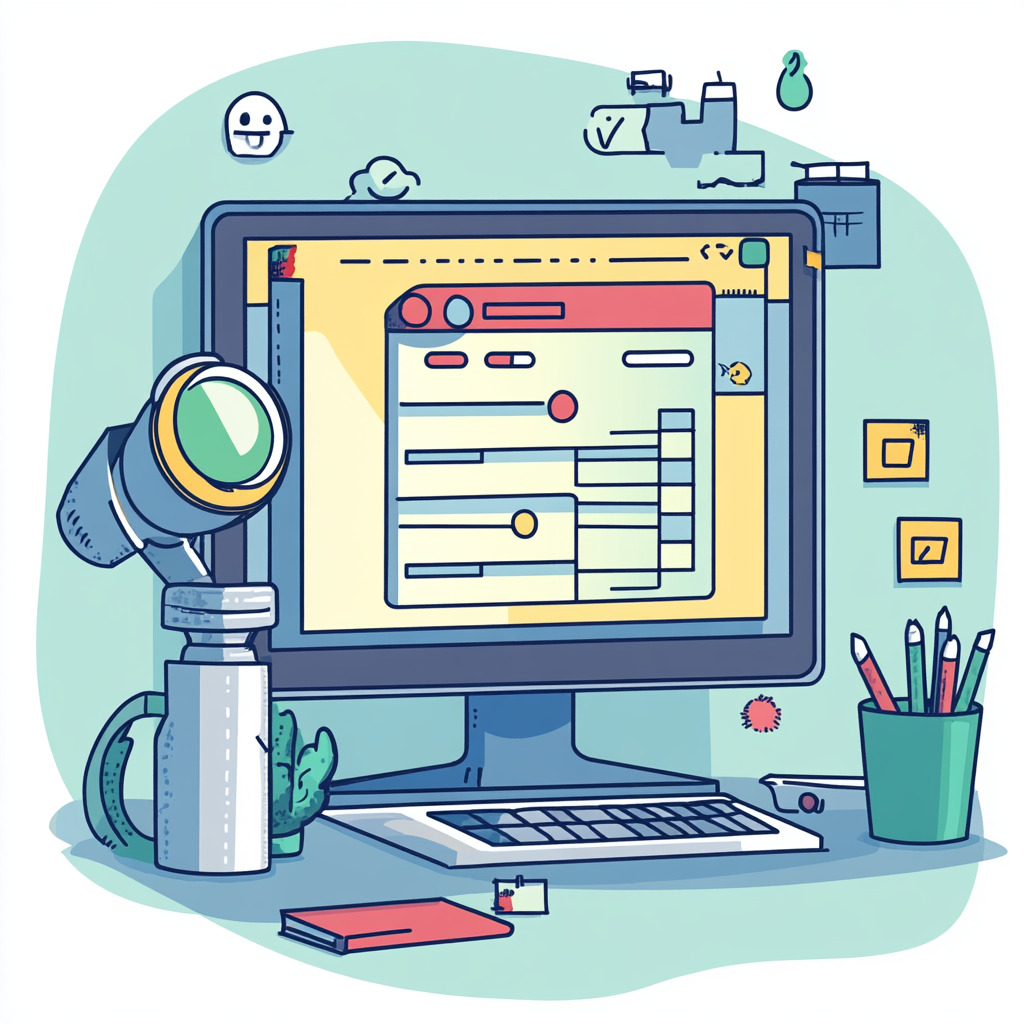
The Many Uses of Laravel Validation: The Power of “Required If”
By Kez | 16 Sep 24
- Introduction to Laravel validation.
- Understanding validation basics.
- Validation rules.
- What is Laravel's "required if" validation rule?
- Scenario: a newsletter signup form.
- Practical Applications of "Required If" Validation Rules
- Dynamic forms.
- Conditional logic based on user roles.
- E-commerce applications.
- Multi-step forms.
- API validation.
- The benefits of using "required if".
- Best practices for using "required if".
- Even more Laravel validation tips.
- Handling validation errors.
- Advanced validation techniques.
- Request validation in Laravel.
- Best practices for validation.
- Laravel and high-quality hosting for agencies.
Laravel’s validation system is one of the most powerful tools for ensuring data integrity and security. For developers working on complex projects, understanding the intricacies of Laravel’s validation rules can greatly enhance the efficiency and reliability of their applications. One extremely useful validation rule is `required_if`. Let’s take a look at the many uses of ‘required_if’ after a quick intro to Laravel validation for anyone who isn’t familiar.
Introduction to Laravel validation.
Laravel validation is a feature-rich component that helps developers validate user inputs effortlessly. It provides a fluent and expressive syntax for defining validation rules. This enables developers to ensure that the data submitted by users on applications is valid before further processing.
Laravel’s validation integrates seamlessly with various input types, such as form submissions, JSON requests, and more, making it versatile for a wide range of applications.
Understanding validation basics.
Validation is the process of checking that data is valid before attempting to use it. This can include checking for required fields, unique values, and data formats.
Validation can be performed on both client-side and server-side, but server-side validation is more secure. Laravel provides a powerful validation system that makes it easy to add validation to applications.
From a client-side perspective, when validation fails, it’s likely that they have missed an input in a form or a required field under validation must be filled in. Custom error messages can be created in this case (as can custom validation messages when fields are filled in correctly). The defined validation rules method is an excellent way of ensuring all fields are filled in and Laravel offers several methods for validating data.
Validation rules.
Laravel provides several handy validation rules, including date, email, and password validation, with easy built-in rules. We’re going to share some of these built-in rules below, but here are some of the things they can affect within apps and on websites:
- Date
- Passwords
- Colour
- Images
- File uploads
- Numerical values
- And any type of required field
However, one of the most useful rules is the ‘required if’ rule – let’s find out more about this valuable tool, shall we?
What is Laravel's "required if" validation rule?
The `required_if` validation rule in Laravel allows you to apply a validation conditionally, based on the value of another field. This means that a particular field will only be required if another specified field has a certain value.
This is particularly useful when dealing with forms or data inputs where the necessity of one input depends on the value or presence of another input. In this case, validation must be present to build a better picture of what the user is trying to accomplish on the website or in the app.
The syntax for using `required_if` is straightforward:
‘field’ => ‘required_if:another_field,value’,
In this rule:
- `field` is the name of the field that should be validated.
- `another_field` is the field whose value is being checked.
- `value` is the value that `another_field` should have for `field` to be required.
Scenario: a newsletter signup form.
Let’s say you’re building a form where users can sign up for a newsletter. It’s a simple form with two fields:
- Subscribe to Newsletter (subscribe) – A checkbox where users can indicate whether they want to receive the newsletter.
- Email Address (email) – A text field where users enter their email address if they want to subscribe.
You want to make the email address field required only if the user checks the “Subscribe to Newsletter” checkbox.
Here’s how the ‘required_if’ validation rule can help:
$request->validate([
‘subscribe’ => ‘boolean’, // This field is optional and can be true or false
’email’ => ‘required_if:subscribe,true|email’, // Email is required only if subscribe is true
]);
Using this validate method to create a form request class like this gives permission to collect data in a database table (in this case, the user’s email address), and send emails to anyone who fills in the form.
“Required if” is simple and highly effective. It’s ideal for creating forms and has a host of other practical applications too.
Practical Applications of "Required If" Validation Rules
Dynamic forms.
One of the most common uses of `required_if` is in dynamic forms, which we briefly covered in the example above. In dynamic forms, certain fields should only be filled out if the user selects or provides a specific answer.
In the example above, the user needs to specify if they want to subscribe to a newsletter. If they tick yes, they are required to input their email address.
Another good example is a job application form. The ‘required_if’ validation rule can be used in many instances on a form like this, but here are a few practical examples:
Let’s say you need to determine whether someone is currently employed, the form will likely have something like this on it:
- Are You Currently Employed? – A dropdown where users select “Yes” or “No.”
- Employer Name – A text field where users enter the name of their current employer if they are employed.
Of course, if a user selects ‘no’, no action will be needed and they can continue on with the rest of the form. If they answer ‘yes’, additional info is needed. Here’s how the validation rule `required_if` can be used:
$request->validate([
‘currently_employed’ => ‘required|in:yes,no’, // Ensures that the field is required and must be either ‘yes’ or ‘no’
’employer_name’ => ‘required_if:currently_employed,yes|string|max:255’, // Required only if currently_employed is ‘yes’
]);
– ‘currently_employed’ => ‘required|in:yes,no’: This rule means that the user must select either “Yes” or “No” in the `currently_employed` dropdown to submit the form. The `in:yes,no` part limits the field to only those two options.
– ’employer_name’ => ‘required_if:currently_employed,yes|string|max:255’: This rule makes the `employer_name` field required only if the `currently_employed` field is “Yes”. It also ensures that the employer name is a string and has a maximum length of 255 characters.
Conditional logic based on user roles.
In many applications, different users have different roles and these roles have different permissions. An admin, for example, may be able to create new users. So, you might want the admin role to provide more information than a standard user. The `required_if` rule can be used to enforce this requirement:
‘admin_code’ => ‘required_if:role,admin|string’,
Here, the user will be required to input their admin code if they have the role of admin and you can make custom messages and custom requirements based on the needs of the application, of course.
E-commerce applications.
E-commerce applications have complex forms for product creation and checkout processes. For example, if a product has a certain attribute, like “on sale,” you might require additional fields like `sale_price`. The `required_if` rule can enforce that `sale_price` must be filled out if the `on_sale` field is true.
‘sale_price’ => ‘required_if:on_sale,true|numeric’,
This rule ensures that a sale price is entered only when the product is marked as being on sale. Rules like this can reduce the likelihood of human error and ensure e-commerce apps run as smoothly as possible.
Multi-step forms.
Multi-step forms are common in web applications where users are guided through a series of steps to complete a task. An HMRC tax return form is a prime example of this. Sometimes, the requirement for certain fields might depend on previous steps. For instance, if a user selects an option in step one that requires additional information in step two, `required_if` can ensure that this additional information is provided.
‘step_two_field’ => ‘required_if:step_one_field,value’,
This ensures that the field in step two is only required if a particular value was selected in step one.
API validation.
APIs often need to validate incoming data to ensure that requests are well-formed and secure. When designing an API that accepts multiple types of requests or payloads, the `required_if` rule can be very helpful. For instance, in an API that supports different content types, you might require different fields depending on the content type specified by the client.
‘json_payload’ => ‘required_if:content_type,application/json|json’,
‘xml_payload’ => ‘required_if:content_type,application/xml|xml’,
This ensures that the correct payload format is provided based on the content type specified by the client.
The benefits of using "required if".
The `required_if` validation rule offers several benefits making it a powerful tool in a Laravel developer’s toolkit:
- Code Simplification – By using `required_if`, you can avoid writing custom validation logic in your controllers or form request classes. This keeps your code cleaner and more maintainable.
- Improved User Experience – Conditional validation rules like `required_if` enhance the user experience by ensuring that users are only asked to provide relevant information. This reduces the risk of errors and frustration and speeds up the process.
- Enhanced Security – By strictly enforcing conditional validation rules, you can prevent malicious users from submitting incomplete or incorrect data, improving the overall security of an application.
- Flexibility – The `required_if` rule offers greater flexibility when handling complex validation scenarios, making it easier to implement dynamic forms, multi-step processes, and role-based logic.
Best practices for using "required if".
While `required_if` is a powerful tool, it’s not a magic tool. It should be used as sparingly as possible to ensure that your validation logic remains clear and maintainable. Here are some best practices to consider:
Keep Validation Rules Readable – When using `required_if`, ensure that your validation rules are easy to understand. If a rule becomes too complex, (which can happen quite quickly) consider refactoring it into a custom validation rule or logic in a form request class.
Test Thoroughly – Conditional validation can introduce edge cases that are not immediately apparent. Be sure to test your validation logic thoroughly, especially when dealing with complex forms or API requests.
Combine with Other Rules – The `required_if` rule can be combined with other validation rules to create more robust validation logic. For example, you can combine it with `string`, `numeric`, or `email` rules to enforce both conditional requirements and data types.
Document Your Logic – When using conditional validation rules, it’s important to document your logic clearly, either in code comments or in your project’s documentation. This ensures that other developers (or even your future self) can easily understand the reasoning behind your validation rules.
Even more Laravel validation tips.
So, that’s the handy ‘required_if’ validation. It’s a great tool to have in your Laravel arsenal, but there are a few other key validation tools that are worth knowing about. So, before we leave you to give ‘required_if’ a try, and tell you about our Laravel hosting service, let’s take a quick look at some more validation tips.
Handling validation errors.
When validation fails, an Illuminate\Validation\ValidationException exception is thrown, and the appropriate error response is returned. The $errors variable in the view file holds all the validation errors. To access errors for a specific field, use $errors->get(‘field’) to get an array of errors. To retrieve the first error message for a field, use $errors->first(‘field’). Use these methods to display validation errors for form fields in your view.
Advanced validation techniques.
Laravel provides another method of writing validations called form requests. A form request is a custom request class that organises validations and declutters the controller. You can define an authorise method in your Form Request class to check if the authenticated user has the authority to update a given resource. If the authorise method returns false, Laravel will automatically abort the request with a 403 Forbidden response. You can customise error messages for validation rules through the messages() method in the request class.
Request validation in Laravel.
To perform request validation in Laravel, you need to create a request class. This class extends the Illuminate\Foundation\Http\FormRequest base class and defines the validation rules for the incoming request. You can generate a new request class using the make:request Artisan command. The validator instance contains two arguments: the data to be validated and an array of validation rules. You can use the Validator facade to validate data manually.
Always validate user input data to prevent security vulnerabilities and data corruption. Use Laravel’s built-in validation rules whenever possible. Create custom validation rules when necessary. Use form request classes to organise validations and declutter controllers. Customise error messages to provide more information to the user. Test your validation rules to ensure they work as expected.
Laravel’s validation system, especially the `required_if` rule, is a critical feature that allows developers to enforce data integrity and improve user experience through conditional logic. It’s an excellent tool to have on hand, and when used sparingly, it’s valuable to app developers, owners and users.
For agencies and developers who rely on Laravel to build sophisticated web applications, understanding and effectively using these validation rules is essential. Still, even the most well-written Laravel applications need a reliable, high-performance hosting environment to survive. That’s where we come in. We’re Nimbus. Partnering with us ensures you have a lightning-fast, dependable, controllable and secure hosting platform to host your and your client’s applications.
Whether you’re running a small portfolio site or a large-scale enterprise application, the right hosting environment can ensure optimal performance, security, and scalability. Get in touch to learn more about our Laravel hosting services and find out why we’re the trusted partner of agencies and developers around the world.