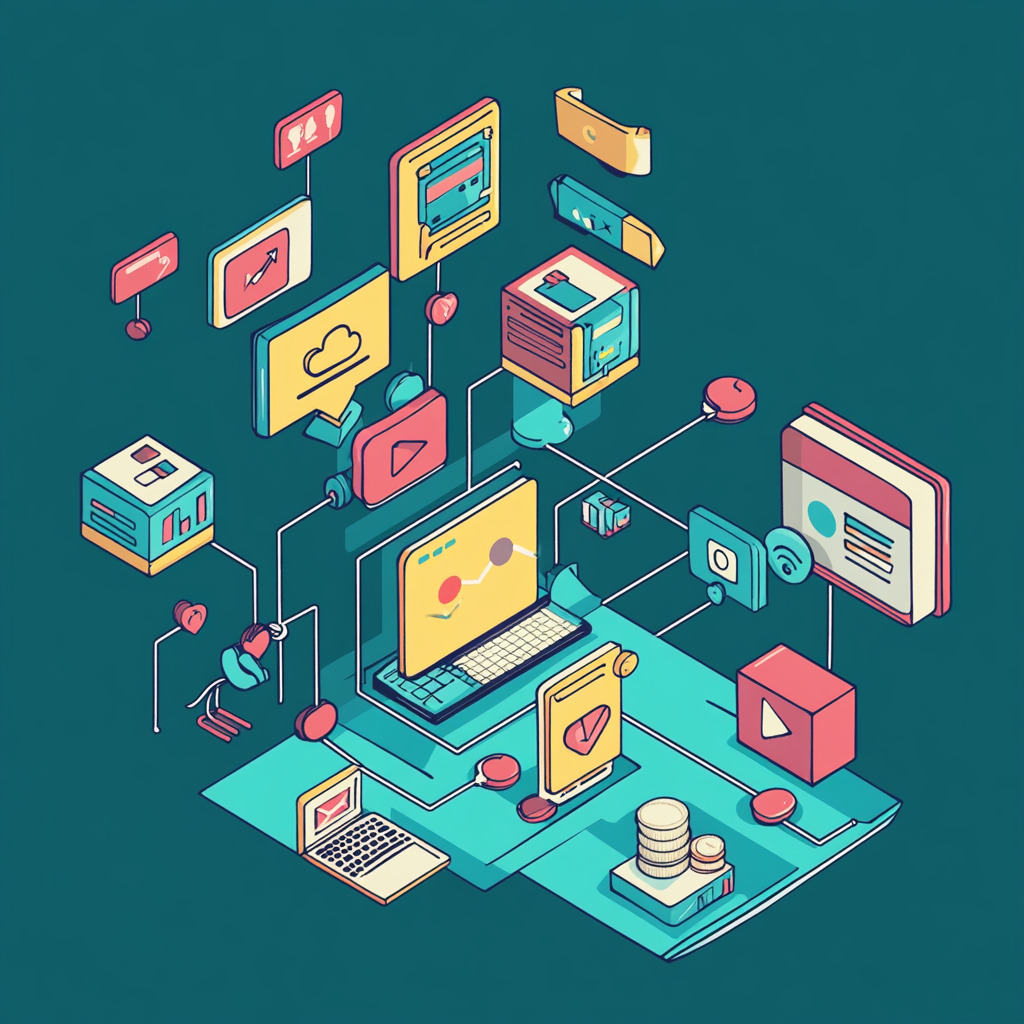
Laravel Migration: Check if Column Exists – Complete Guide
By John | 04 Oct 24
Laravel’s migration system is one of its most powerful features. It allows you to manage all of your database schemas easily. Sometimes, you might need to check if a particular column exists in a table before performing a migration to avoid errors, duplications or redundant changes. But how do you check if a column exists in Laravel? Well, here’s a complete guide. Oh, and if you need hosting for your Laravel sites and apps, stick around because we offer powerful Laravel hosting packages that you’ll want to explore!
Why Check for Column Existence?
When working on a shared codebase or maintaining an application with multiple environments, you may encounter situations where a column is already present in some environments but missing in others. Running migrations that blindly add or modify columns in an existing table without checking for their existence can lead to SQL errors and a few headaches, to say the least.
For instance, running a migration that tries to add a column that already exists would throw an error like this:
SQLSTATE[42S21]: Column already exists: 1060 Duplicate column name ‘your_column’ (SQL: alter table `your_table` add `your_column` …
To prevent these issues, Laravel provides a simple way to check if a column exists before performing any operations on it and it works in a user table, database table, flights table and loads more. So, before you create a migration file, here’s how to check if a column exists.
Using `hasColumn` to Check for Column Existence
Laravel’s `Schema` facade includes a method called `hasColumn` that lets you easily check if a column exists in a table. Here’s how to use it in a migration:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddYourColumnToYourTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
if (!Schema::hasColumn(‘your_table’, ‘your_column’)) {
Schema::table(‘your_table’, function (Blueprint $table) {
$table->string(‘your_column’)->nullable();
});
}
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
if (Schema::hasColumn(‘your_table’, ‘your_column’)) {
Schema::table(‘your_table’, function (Blueprint $table) {
$table->dropColumn(‘your_column’);
});
}
}
}
How It Works:
If you aren’t familiar with Laravel code, while it’s beautifully simple for the initiated, it can be tricky to see what’s happening, so here’s a breakdown:
`Schema::hasColumn()` – This method takes two arguments—the table name and the column name—and returns `true` if the column exists and `false` otherwise.
`if (!Schema::hasColumn())` – This condition ensures that the new column is only added if it doesn’t already exist. This prevents the migration from failing if the column is already present.
`$table->dropColumn()` – Similarly, when rolling back the migration in the `down()` method, we first check if the column exists before attempting to drop it.
Put very simply, this migrate artisan command ensures that if a column exists in one instance, it’s added to another. So you can create migration with confidence and far fewer headaches! Here are some more handy tips for handling column migrations.
Want to check which Laravel version an app or site using? We have a guide for that too!
Handling Multiple Columns
If you need to check for multiple columns, you can extend the logic like so:
if (!Schema::hasColumns(‘your_table’, [‘first_column’, ‘second_column’])) {
Schema::table(‘your_table’, function (Blueprint $table) {
$table->string(‘first_column’)->nullable();
$table->integer(‘second_column’)->default(0);
});
}
Unfortunately, Laravel doesn’t offer a built-in `hasColumns` method, but you can handle multiple checks with an array:
if (!Schema::hasColumn(‘your_table’, ‘first_column’) && !Schema::hasColumn(‘your_table’, ‘second_column’)) {
Schema::table(‘your_table’, function (Blueprint $table) {
$table->string(‘first_column’)->nullable();
$table->integer(‘second_column’)->default(0);
});
}
Conclusion
When working with Laravel migrations, checking if a column exists before making changes is always a good idea and good practice. Using `Schema::hasColumn()` helps prevent migration failures and ensures that your database schema is updated smoothly across all environments. By adding these checks, you can make your migrations more robust and handle existing database structures gracefully.
So, now you know how to migrate columns in Laravel, why not migrate your Laravel applications and websites over to a powerful, secure and dependable Nimbus server? We provide VPS and dedicated servers for digital agencies and developers and have dedicated features for Laravel users that ensure your applications work seamlessly. Find out more by checking out our Laravel Hosting packages, or book a tour of our platform to see the power for yourself!