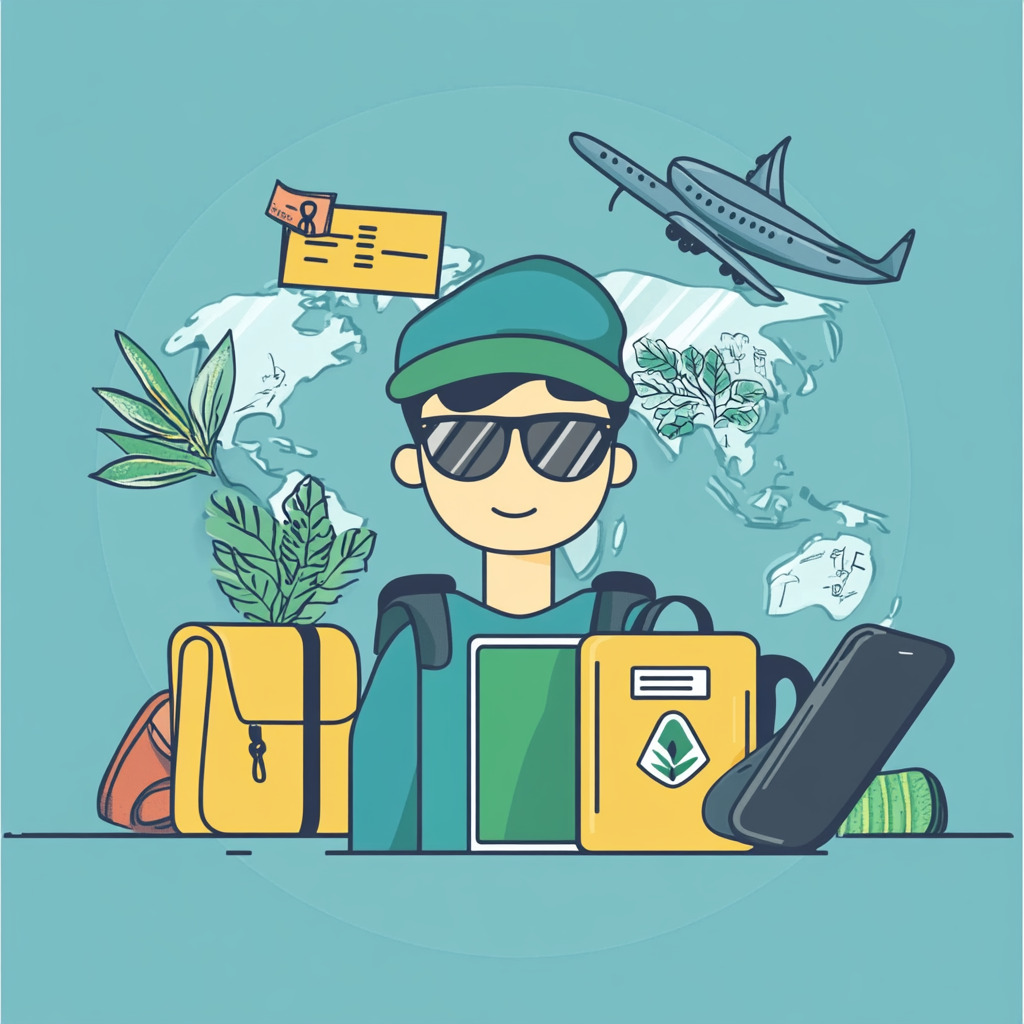
Implementing user authentication using Laravel Passport
By John | 19 Nov 24
- How to implement user authentication using Laravel Passport
- Why Laravel Passport?
- Laravel's core authentication system vs. Laravel Passport
- Setting up Laravel Passport.
- Configuring API authentication with Passport.
- Generating and using access tokens.
- Managing scopes and permissions.
- Testing your passport authentication.
- Refreshing and revoking tokens.
- In conclusion
Authentication is at the heart of any secure web application, especially when handling user data. Laravel provides multiple options for implementing authentication, but for APIs, Laravel Passport is the best solution. It allows for OAuth2 authentication, enabling users to securely access resources while ensuring their credentials remain safe.
In this guide, we’ll take you through implementing user authentication using Laravel Passport, covering everything from installation to securing your API endpoints with access tokens. By the end, you’ll be able to set up a secure authentication layer that protects your Laravel application.
How to implement user authentication using Laravel Passport
Why Laravel Passport?
Before we dive into the steps, you might be wondering why using Laravel Passport is the right option for API authentication. Well, here’s why we’d recommend it:
OAuth2 Protocol – Passport implements the OAuth2 standard which provides a secure solution for managing access tokens.
Simple setup – With Passport, adding API authentication to your Laravel application is really straightforward and well-documented.
Comprehensive features – It offers everything from access tokens and scopes to a built-in UI for token management.
Secured access – Laravel Passport ensures that all API endpoints are protected, giving users secure access to their data while preventing unauthorised access.
Laravel's core authentication system vs. Laravel Passport
Passport uses Laravel’s core Authenticatable class as a base for authentication and then adds advanced API-specific functionality, making it ideal for building secure, token-based API authentication. Here’s how they work together:
Core Authentication (Authenticatable) – The class ‘user extends authenticatable’ enables basic authentication features for the User model, handling traditional session-based authentication (e.g., logging in via a web form). This is what powers the basic Auth::attempt() method and provides support for the auth:api middleware.
Passport’s Extension – Passport leverages the foundational authentication features provided by Authenticatable but introduces token-based authentication, specifically for APIs using the OAuth2 protocol. When you install Passport, it adds extra methods to the User model, such as createToken() for generating tokens. Passport also provides middleware and guards to protect API routes using access tokens instead of sessions.
Setting up Laravel Passport.
Laravel Passport is an excellent option for authenticating incoming API requests and is much more secure than the core offering from Laravel. So, let’s get it installed and configured, shall we?
Step 1: Install passport.
First, install Passport via Composer:
composer require laravel/passport
After installation, run the Passport installation command:
php artisan passport:install
This command generates the encryption keys required to generate secure access tokens and store them.
Step 2: Configure the service provider.
Next, open the `config/app.php` file and register the Passport service provider. This should already be done in Laravel 10+, but confirm it’s present:
‘providers’ => [
// Other service providers…
Laravel\Passport\PassportServiceProvider::class,
],
Then, use Passport’s routes by calling `Passport::routes` in the `AuthServiceProvider`:
use Laravel\Passport\Passport;
public function boot()
{
$this->registerPolicies();
Passport::routes();
}
Step 3: Run migrations.
Laravel Passport comes with migrations to create the necessary tables for storing clients, access tokens, and more. Run the following command to set up your database:
php artisan migrate
With these migrations in place, your Laravel application now has tables for managing clients and tokens.
Configuring API authentication with Passport.
Once Passport is installed and configured, you need to set up authentication for your API routes.
Step 1: Update the auth config.
In `config/auth.php`, update the `api` driver to `passport`:
‘guards’ => [
‘api’ => [
‘driver’ => ‘passport’,
‘provider’ => ‘users’,
],
],
This setting tells Laravel to use Passport’s access token-based authentication for API routes.
Step 2: Protect API routes.
To secure routes, use Laravel’s `auth:api` middleware. Open the `routes/api.php` file and add `auth:api` middleware to the routes that require authentication:
Route::middleware(‘auth:api’)->get(‘/user’, function (Request $request) {
return $request->user();
});
Only authenticated users with valid tokens can now access these routes.
Generating and using access tokens.
With Passport installed, your application can now generate access tokens and issue them to users. Here’s how to handle registration, login, and token management.
Step 1: Register and login users.
Create an `AuthController` to handle registration and login logic. In the `register` method, create a new user and issue an access token. In the `login` method, validate credentials and issue a token if they are correct.
use Illuminate\Http\Request;
use App\Models\User;
use Illuminate\Support\Facades\Auth;
class AuthController extends Controller
{
public function register(Request $request)
{
$validatedData = $request->validate([
‘name’ => ‘required|string|max:255’,
’email’ => ‘required|string|email|max:255|unique:users’,
‘password’ => ‘required|string|min:8|confirmed’,
]);
$user = User::create([
‘name’ => $validatedData[‘name’],
’email’ => $validatedData[’email’],
‘password’ => bcrypt($validatedData[‘password’]),
]);
$token = $user->createToken(‘AuthToken’)->accessToken;
return response()->json([‘token’ => $token], 201);
}
public function login(Request $request)
{
$credentials = $request->only(’email’, ‘password’);
if (Auth::attempt($credentials)) {
$token = Auth::user()->createToken(‘AuthToken’)->accessToken;
return response()->json([‘token’ => $token], 200);
} else {
return response()->json([‘error’ => ‘Unauthorized’], 401);
}
}
}
Registration – Validates input, creates the user, and generates a token.
Login – Authenticates credentials and, if successful, issues a token.
Step 2: Use access tokens in API requests.
Once users have their tokens, they can use them in subsequent API requests. For secured routes, include the token in the `Authorization` header:
Authorization: Bearer {access_token}
Laravel will automatically verify the token and grant access if it’s valid.
Managing scopes and permissions.
Passport allows you to define scopes. These are permissions that determine what actions a token holder can perform.
Step 1: Define scopes in `AuthServiceProvider`.
In `AuthServiceProvider`, define the scopes your application requires:
Passport::tokensCan([
‘view-posts’ => ‘View blog posts’,
‘manage-posts’ => ‘Create, update, delete blog posts’,
]);
Step 2: Assign scopes when creating tokens.
You can also specify scopes when generating tokens in your login or register functions:
$token = $user->createToken(‘AuthToken’, [‘view-posts’])->accessToken;
Step 3: Check scopes in middleware.
Use the `scope` middleware to ensure that only users with the right permissions can access specific routes:
Route::middleware([‘auth:api’, ‘scope:view-posts’])->get(‘/posts’, function () {
return response()->json([‘message’ => ‘Access granted to view posts’]);
});
If a user tries to access a route without the required scope, they’ll receive a `403 Forbidden` response.
Testing your passport authentication.
With any PHP file changes, testing ensures that your setup works as expected. Here’s how to test Laravel Passport changes:
Unit tests – Test registration, login, and token generation using PHPUnit or Laravel’s built-in testing capabilities.
API testing – Use tools like Postman or Curl to test API endpoints with and without access tokens to confirm that Passport correctly grants or denies access.
Refreshing and revoking tokens.
Passport automatically handles token expiration, but you can manage the token lifecycle for additional control.
Refresh tokens – Laravel Passport supports refresh tokens out-of-the-box to maintain secure sessions.
Revoking tokens – Use Passport’s built-in token revocation methods to log users out or restrict access.
In conclusion
Laravel Passport provides a strong solution for implementing user authentication in API-driven applications. It’s easy to set up, has built-in support for OAuth2 and advanced options like scopes and token management. So, it’s perfect for developers building secure, scalable applications.
As are our Laravel hosting options. Whether you’re a freelance developer or part of an agency looking for a secure, powerful hosting provider, we can help. Our Laravel hosting packages offer the ultimate VPS or dedicated servers for developers who need unlimited storage for their client’s apps and websites.
Explore our pricing packages or take a platform tour to see why so many Laravel developers have already made the switch to Nimbus.