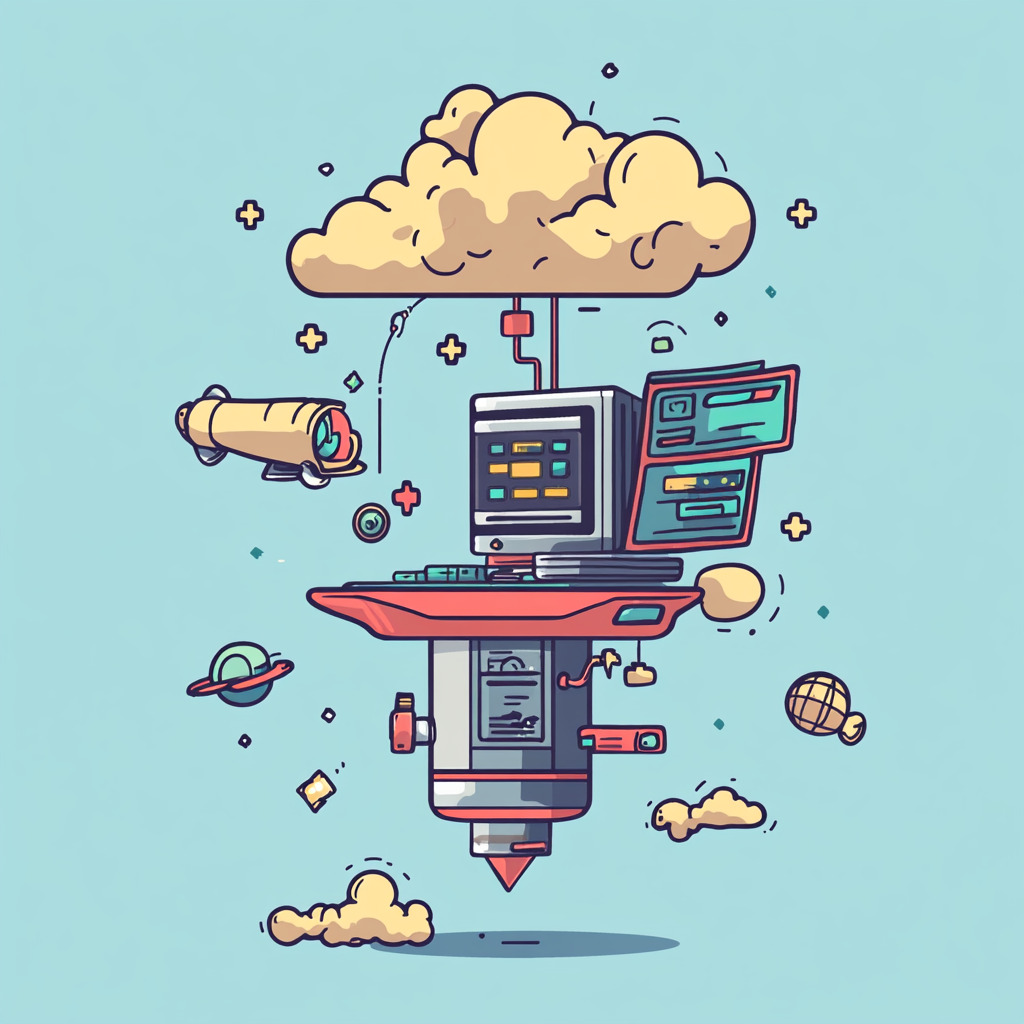
How to Perform a Laravel Cache Clear: A Comprehensive Guide
By Kez | 09 Sep 24
- Understanding Laravel's caching system.
- Why clear cache?
- When not to clear cache in Laravel.
- How to clear cache in Laravel.
- 1. Clearing application cache.
- 2. Clearing route cache.
- 3. Clearing configuration cache.
- 4. Clearing view cache.
- 5. Clearing event cache.
- 6. Clearing cache programmatically.
- Best practices for managing cache.
- Conclusion.
Among its many features, Laravel’s caching system plays a crucial role in optimising performance by reducing the load on the database and speeding up the application’s response time. However, there are times when you need to clear the cache – like after updating the code, changing configuration files, or during the deployment process, for example. Let’s take a look at the various methods of performing a cache clear in Laravel, to ensure your applications run smoothly.
Understanding Laravel's caching system.
Before diving into the methods of clearing cache in Laravel, let’s look at how Laravel handles caching. Laravel supports multiple cache drivers, such as `file`, `database`, `memcached`, `redis`, and more. By default, Laravel uses the `file` driver, which stores cached data in the `storage/framework/cache` directory.
Caching in Laravel can be categorised into several types:
- Config Cache – Stores all configuration values.
- Route Cache – Stores route definitions.
- View Cache – Stores compiled Blade templates.
- Application Cache – Stores various cached data, like query results, third-party service responses, etc.
Understanding Laravel cache usage is really important to ensure you aren’t removing vital data or a key value that your app needs to run correctly.
Why clear cache?
Clearing an entire cache driver is necessary in several scenarios:
After Deployment – When deploying updates, especially configuration changes, it’s essential to clear the cache to ensure the application uses the latest settings.
Debugging – Sometimes, cached data might cause unexpected behaviour during development. Clearing the cache data can help resolve such issues.
Performance Tuning – Regularly clearing cache can prevent the accumulation of stale data, which can slow down the application over time.
When not to clear cache in Laravel.
In Laravel applications, you should avoid clearing the cache in production environments unless absolutely necessary. The cache is critical for performance optimisation, as it stores compiled views, configuration files, and route information, all of which reduce the need to recompute them on each request.
Clearing the cache can lead to a temporary performance hit, increased server load, and potential user experience disruptions. It might also expose race conditions or bugs masked by the cached data.
So, only clear the cache when deploying significant changes, troubleshooting specific issues, or if a specific update requires you to clear the cache.
How to clear cache in Laravel.
Laravel provides a set of artisan commands to clear different types of cache. Let’s explore each of them, shall we?
1. Clearing application cache.
The application cache contains various data like query results, third-party API responses, and custom data that you might cache during development. To clear the application cache, use the following command:
php artisan cache:clear
2. Clearing route cache.
Route caching is a great way to speed up route registration by caching the entire route configuration. However, if you modify your routes, you need to clear the route cache to apply those changes. Use the following command:
php artisan route:clear
To re-cache the routes after clearing, you can use:
php artisan route:cache
This will regenerate the route cache file.
3. Clearing configuration cache.
Configuration caching is another performance enhancement feature in Laravel. It compiles all of your configuration files into a single file, which is then loaded quickly by the framework. If you change any configuration values or environment variables, clear the configuration cache using:
php artisan config:clear
After clearing, run this cache command to regenerate the configuration cache:
php artisan config:cache
4. Clearing view cache.
Laravel compiles Blade templates into plain PHP code and caches them for faster rendering. If you modify a Blade template and notice that the changes aren’t reflecting, you’ll need to clear the view cache with the following artisan command:
php artisan view:clear
This will delete all cached Blade files from the storage directory.
5. Clearing event cache.
In Laravel, event caching stores a manifest of all events and listeners, which can be cleared with the following command:
php artisan event:clear
If needed, you can rebuild the event cache using:
php artisan event:cache
6. Clearing cache programmatically.
In some cases, you might want to clear the cache programmatically from within your application, perhaps in a controller or middleware. You can do this by using the `Cache` facade:
use Illuminate\Support\Facades\Cache;
Cache::flush();
This method will clear all cache items across the default cache driver and all other cache stores, which can be useful if you need to clear the cache based on certain conditions.
Best practices for managing cache.
Avoid Clearing Cache Frequently – While clearing the cache can solve plenty of issues, doing it too often can degrade performance since the application needs to regenerate cached data so it should be done responsibly. For example, clearing the cache in Laravel after setting up a PHP namespace app, ensures that any conflicts within the file cache driver are removed.
Use Cache Wisely – Cache only the data that is expensive to retrieve or compute. Over-caching can lead to stale data issues.
Automate Cache Clearing – Consider integrating cache-clearing commands into your deployment pipeline. This ensures the application always runs with the most recent configurations and routes without manual intervention.
Conclusion.
Laravel’s caching system is a powerful tool that, when used effectively, can significantly enhance your application’s performance. Still, it’s important to know how to manage and clear caches wisely to avoid issues that arise from stale data. By using the artisan commands mentioned above, you can keep your Laravel application running smoothly and efficiently.
Whether you’re a seasoned Laravel developer or just starting, understanding how to clear the cache in Laravel is a vital skill that will help you maintain your application’s performance and reliability. Do you know what else will maintain your application’s performance? Lightening fast, secure and reliable hosting from Nimbus! We specialise in Laravel hosting for agencies and developers. Learn more on our Laravel hosting and PHP hosting pages, or speak with our team today to take control of your hosting and ensure your apps are as powerful as possible!