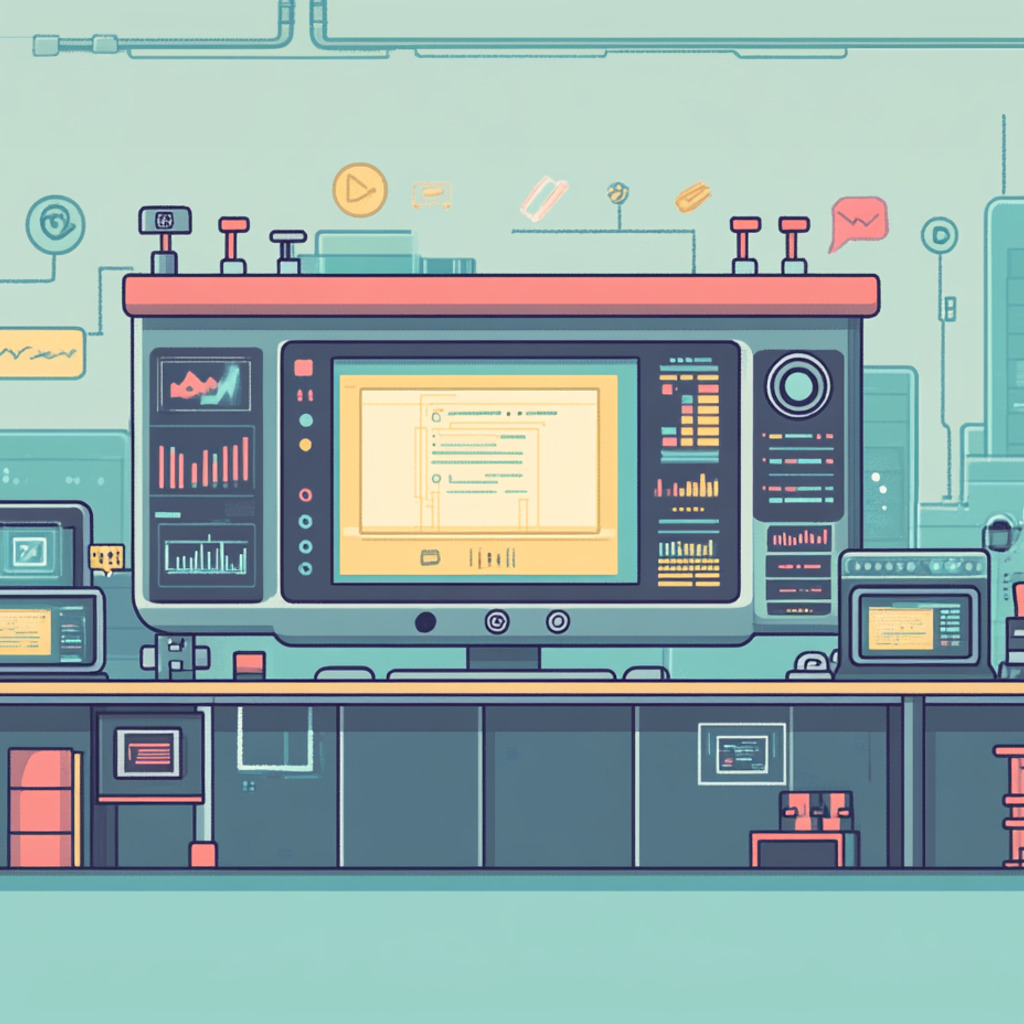
Everything you need to know about Laravel migration rollback
By John | 18 Oct 24
- How Laravel migration rollbacks work.
- Key rollback commands for a database migration.
- 1. `php artisan migrate:rollback`
- 2. `php artisan migrate:reset`
- 3. `php artisan migrate:refresh`
- 4. `php artisan migrate:fresh`
- Level up your Laravel hosting plan with Nimbus!
- Practical tips for rollbacks.
- 1. Always define the `down()` method.
- 2. Use transactions for complex rollbacks.
- 3. Be cautious when rolling back in production.
- 4. Testing rollbacks locally before deployment.
- Advanced usage scenarios of rollback migration.
- Rolling back a specific migration.
- Rolling back with seeder data.
- The most important thing!
- Ready to take your Laravel hosting to the next level?
In any good Laravel application, database migrations play a crucial role in managing schema changes over time. Adding tables, columns or constraints to your Laravel project is a great way of moving it forward, but sometimes we need to go backwards – this is where the Laravel migration rollback command comes in.
Laravel makes rolling back your latest migration seamless. Still, there’s more to it than just running a single command – we wish it were that simple. In this post, we’ll cover everything from the basics of rollback to more advanced scenarios. There are plenty of practical tips below too. So, whether you’ve never done database migrations or have multiple migration files to manage, we have some info below to help you.
How Laravel migration rollbacks work.
A migration rollback in Laravel allows you to reverse the most recent or a specific number of database migrations that have been applied. This feature is particularly handy when you’ve made a mistake in your migrations, or you want to undo a series of migrations for testing purposes.
Rollbacks are particularly useful in environments like local development or staging, where you may want to iterate on your database structure without impacting production.
Laravel manages migrations through the `migrations` table in your database. Each migration is recorded here with a unique timestamp. When you run a rollback, Laravel reads from the existing database tables to determine which migrations to reverse and applies the corresponding down methods.
To define how the rollback should behave, each migration file contains two methods:
- – `up()`: Executes the migration.
- – `down()`: Reverses the migration.
The `down()` method is executed when rolling back. If you don’t define this method, Laravel will throw an error when you attempt to roll back the migration.
Key rollback commands for a database migration.
Laravel provides several commands to help manage rollbacks at different levels of granularity. Which one you choose to use depends on how far back in time you want to roll back. Here are the most commonly used ones:
1. `php artisan migrate:rollback`
This command rolls back the last migration batch found in the existing table. A “batch” in Laravel refers to a group of migrations that were run in the same instance of the `php artisan migrate` command.
php artisan migrate:rollback
By default, it rolls back one batch. However, you can specify the number of batches you wish to rollback using the `–step` option.
php artisan migrate:rollback –step=2
This will rollback the last two batches of migrations.
2. `php artisan migrate:reset`
While `rollback` only undoes the last batch, `reset` will undo all migrations applied, regardless of when they were applied.
php artisan migrate:reset
This command effectively re-creates an un-migrated state. It’s useful when you want to completely reset your database to an un-migrated state.
3. `php artisan migrate:refresh`
The `refresh` command line rolls back all migrations and then runs them again. This effectively rebuilds your database from scratch. This is handy when you want to quickly test migrations without having to manually rollback and re-run.
php artisan migrate:refresh
The method above will refresh all migration files on the entire database. You can also add the `–step` option here if you only want to rollback and re-run a specific number of migrations:
php artisan migrate:refresh –step=1
4. `php artisan migrate:fresh`
The `fresh` command drops all tables and then re-runs all migrations from scratch. Unlike `refresh`, it doesn’t roll back the migrations – it simply drops the tables and runs the `up()` methods again.
php artisan migrate:fresh
This rollback method is useful when you want to make sure that there are no lingering data or schema issues from a previous state.
Level up your Laravel hosting plan with Nimbus!
Do you need powerful and reliable Laravel hosting for your agency? If you’re a Laravel developer, host your websites and apps in a hosting environment you can trust with Nimbus. With VPS and dedicated server options, your websites and apps will be secure, fast and always perform at their best.
Get in touch today to find out why we’re a trusted partner to so many digital agencies and freelancers around the UK.
Practical tips for rollbacks.
Let’s dive into some more practical tips that can help you avoid headaches when rolling back migrations.
1. Always define the `down()` method.
If you forget to define the `down()` method in your migrations, Laravel won’t know how to reverse the migration – and this will throw up an error code. To avoid this, and as a best practice, always write the `down()` method, even if it’s as simple as dropping a column or table.
Example of a migration with a defined `down()` method:
public function up()
{
Schema::create(‘posts’, function (Blueprint $table) {
$table->id();
$table->string(‘title’);
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists(‘posts’);
}
Without a `down()` method, you may need to manually reverse the migration by crafting a new migration or executing raw SQL. As any seasoned Laravel developer knows, this is time-consuming and prone to errors. So, we’d recommend taking the easy option wherever possible.
2. Use transactions for complex rollbacks.
For complex migrations involving multiple tables or operations, it’s a good idea to wrap your migration in a database transaction. This ensures that either all migration steps are applied, or none are, making it easier to rollback cleanly in case of failure.
You can use the `DB::transaction()` method in your migration files like this:
public function up()
{
DB::transaction(function () {
// Perform multiple operations that should all succeed or fail as one unit
});
}
public function down()
{
DB::transaction(function () {
// Rollback logic here
});
}
3. Be cautious when rolling back in production.
Rolling back migrations in production can be risky, especially if you’re dealing with large datasets, complex relationships, or critical business data. So, this likely goes without saying, always back up your production database before rolling back. If you’re using a Nimbus hosting plan for your Laravel sites, taking a backup is really simple and takes a matter of seconds.
Additionally, it’s wise to keep migrations as atomic as possible in production. If your migration introduces a breaking change or requires downtime, consider using zero-downtime deployment strategies like chunking updates or creating new tables instead of modifying existing ones directly.
4. Testing rollbacks locally before deployment.
Before pushing your migrations to production, it’s a good idea to test the entire lifecycle of the migration, including rollbacks, on your local or staging environment. Run `php artisan migrate`, make sure your schema is correct, and then run `php artisan migrate:rollback` to verify that the rollback works as expected. Again, if you have Laravel hosting with Nimbus, this is nice and easy to do.
If you’re using version control (like Git), you can leverage branches to test different migration states without affecting the main branch.
Advanced usage scenarios of rollback migration.
Rolling back a specific migration.
By default, Laravel rolls back migrations by batch, but sometimes, you may need to roll back a specific migration, say, one from a few batches ago. Unfortunately, Laravel doesn’t provide an out-of-the-box solution for rolling back a single migration that wasn’t part of the last batch – at least at the time of writing.
However, you can achieve this by creating a new migration to manually undo the changes from that specific migration. This allows you to reverse a single migration without affecting others.
Rolling back with seeder data.
Often, migrations are paired with seeders to populate tables with initial data. If you’re rolling back a migration, you might also want to remove or update the seeded data. In this case, you can combine rollbacks with the `db:seed` command or custom logic inside your seeders.
For example, after a rollback, you might want to re-seed the database:
php artisan migrate:rollback && php artisan db:seed
Or, in more complex scenarios, you could create conditional seeding logic to ensure that the database state is consistent after a rollback.
The most important thing!
Laravel’s migration rollback feature is a powerful tool for managing your database schema, especially during development. It allows you to iterate quickly, fix mistakes, and ensure your schema evolves smoothly. However, with great power comes great responsibility – so, we’ll reiterate a point from above before we leave you to your coding – always, always, take a backup, define your `down()` methods, and test thoroughly.
Ready to take your Laravel hosting to the next level?
As we mentioned earlier, we’re Nimbus. We offer robust, secure and lightning-fast hosting for Laravel and other PHP websites and applications. If you need hosting for your agency, or your side projects, check out our hosting plans before you leave. Or for more information about our fast, reliable and secure platform, get in touch to take a platform tour today!